TXID
Transaction ID
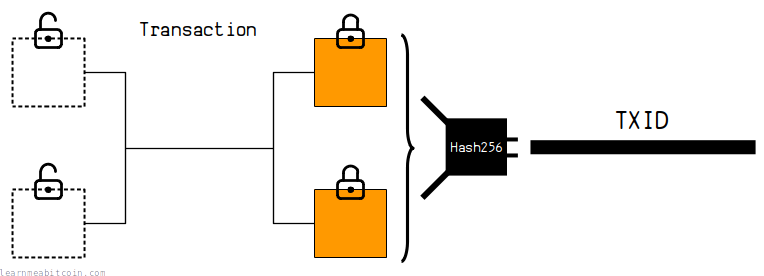
A TXID (Transaction ID) is a unique reference for a bitcoin transaction.
They're used to look up specific transactions in a blockchain explorer. For example:
- f4184fc596403b9d638783cf57adfe4c75c605f6356fbc91338530e9831e9e16 — First ever Bitcoin transaction to Hal Finney in 2009.
- a1075db55d416d3ca199f55b6084e2115b9345e16c5cf302fc80e9d5fbf5d48d — Pizza transaction for 10,000 BTC in 2010.
- 4ce18f49ba153a51bcda9bb80d7f978e3de6e81b5fc326f00465464530c052f4 — The transaction containing the first donation I received for making this website.
The letters and numbers in a TXID have no special meaning. They're just random-looking bunches of 32 bytes (represented as 64 hexadecimal characters). But they are unique to each transaction.
Creating
How do you create a TXID?
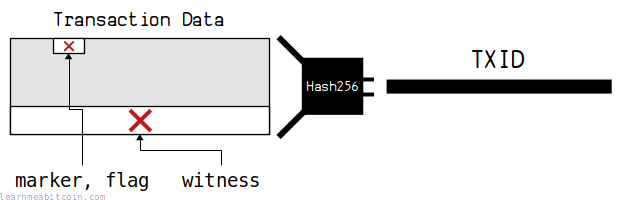
A TXID is created by hashing the transaction data. More precisely, it's created by putting specific parts of the transaction data through the SHA256 hash function, then putting the result through the SHA256 again (this double-SHA256 hashing is referred to as HASH256).
- For legacy transactions you HASH256 all of the transaction data.
- For segwit transactions you HASH256 all of the transaction data except the marker, flag, witness fields.
So for segwit transactions the signatures are no longer included as part of the TXID.
The TXIDs you see on blockchain explorers are actually in reverse byte order. This is just a quirk of bitcoin.
Code
A TXID is created in the same way as a block hash. You just need to HASH256 the correct parts of transaction data to create the TXID:
require 'digest'
# ----------------
# transaction data
# ----------------
data = "0100000001c997a5e56e104102fa209c6a852dd90660a20b2d9c352423edce25857fcd3704000000004847304402204e45e16932b8af514961a1d3a1a25fdf3f4f7732e9d624c6c61548ab5fb8cd410220181522ec8eca07de4860a4acdd12909d831cc56cbbac4622082221a8768d1d0901ffffffff0200ca9a3b00000000434104ae1a62fe09c5f51b13905f07f06b99a2f7159b2225f374cd378d71302fa28414e7aab37397f554a7df5f142c21c1b7303b8a0626f1baded5c72a704f7e6cd84cac00286bee0000000043410411db93e1dcdb8a016b49840f8c53bc1eb68a382e97b1482ecad7b148a6909a5cb2e0eaddfb84ccf9744464f82e160bfa9b8b64f9d4c03f999b8643f656b412a3ac00000000"
# ----
# TXID
# ----
# Note: Don't put the transaction data into the hash function as a string.
# Convert it from hexadecimal to raw bytes first.
# convert hexadecimal string to byte sequence
bytes = [data].pack("H*") # H = hex string (highest byte first), * = multiple bytes
# SHA-256 (first round)
hash1 = Digest::SHA256.digest(bytes)
# SHA-256 (second round)
hash2 = Digest::SHA256.digest(hash1)
# convert from byte sequence back to hexadecimal string
txid = hash2.unpack("H*")[0]
# print result (natural byte order)
puts txid #=> 169e1e83e930853391bc6f35f605c6754cfead57cf8387639d3b4096c54f18f4
# print result (reverse byte order)
puts txid.scan(/../).reverse.join #=> f4184fc596403b9d638783cf57adfe4c75c605f6356fbc91338530e9831e9e16
Remember that when working with segwit transactions you do not include the marker, flag, and witness as part of the transaction data that is being hashed.
Examples
What does a TXID look like?
1. Legacy Transaction
To create a TXID for a legacy transaction you HASH256 all of the transaction data:
0100000001c997a5e56e104102fa209c6a852dd90660a20b2d9c352423edce25857fcd3704000000004847304402204e45e16932b8af514961a1d3a1a25fdf3f4f7732e9d624c6c61548ab5fb8cd410220181522ec8eca07de4860a4acdd12909d831cc56cbbac4622082221a8768d1d0901ffffffff0200ca9a3b00000000434104ae1a62fe09c5f51b13905f07f06b99a2f7159b2225f374cd378d71302fa28414e7aab37397f554a7df5f142c21c1b7303b8a0626f1baded5c72a704f7e6cd84cac00286bee0000000043410411db93e1dcdb8a016b49840f8c53bc1eb68a382e97b1482ecad7b148a6909a5cb2e0eaddfb84ccf9744464f82e160bfa9b8b64f9d4c03f999b8643f656b412a3ac00000000
Note: The data that gets hashed to create the TXID is highlighted in green.
If you HASH256 all of this data you get 169e1e83e930853391bc6f35f605c6754cfead57cf8387639d3b4096c54f18f4
, which is the TXID in natural byte order and is what's found inside raw transaction data.
Then, if you reverse the byte order you get the TXID f4184fc596403b9d638783cf57adfe4c75c605f6356fbc91338530e9831e9e16, which is the byte order used when searching for transactions in blockchain explorers.
2. Segwit Transaction
To create a TXID for a segwit transaction you HASH256 all of the transaction data except the marker, flag, and witness fields.
020000000001013a53de6e1fe821452674c5435e3989eecdf35cb1de1c8bafb674f543a55d658c3600000000fdffffff01599aea0400000000160014cfbd92a6337e8b6043552d6fc5c35c7e5062281e0247304402201250febbce0a5b333c2d715b869cb960f5abf1702192c7af6e112c6d6030be880220073c55f4814a064bf804d9ed16b57eaaeaafb536c4187e6260ef3fc61ca98a77012102e71911951e1f9799d5ccd05200ea0c18f786cb1bb45754d4a0799a06c2b80e8000000000
Note: The data that gets hashed to create the TXID is highlighted in green.
If you HASH256 the highlighted data you get 01cda497b58d876f207b74c1f0b741f397c376852b3c68b0b6db042a24ffd96c
, then if you reverse the byte order you get the TXID 6cd9ff242a04dbb6b0683c2b8576c397f341b7f0c1747b206f878db597a4cd01.
When creating a TXID for a segwit transaction, you do not hash the fields that are new to segwit transactions. This avoids having any signature data forming part of the TXID (which are now in the witness instead) as signatures can be manipulated to change the TXID after a transaction has been sent into the network (which is rare, but it makes TXIDs less dependable).
This was the primary reason for the segregated witness upgrade.
Try it yourself
You can check that the above data produces the correct TXIDs by manually hashing the same data using HASH256 directly:
Then don't forget to reverse the byte order:
Usage
How are TXIDs used in Bitcoin?
TXIDs play an important role in the way Bitcoin works. They are used in the following situations:
1. Searching for transactions
You typically use TXIDs to look up specific transactions on a blockchain explorer or from your own local node:
$ bitcoin-cli getrawtransaction f4184fc596403b9d638783cf57adfe4c75c605f6356fbc91338530e9831e9e16
0100000001c997a5e56e104102fa209c6a852dd90660a20b2d9c352423edce25857fcd3704000000004847304402204e45e16932b8af514961a1d3a1a25fdf3f4f7732e9d624c6c61548ab5fb8cd410220181522ec8eca07de4860a4acdd12909d831cc56cbbac4622082221a8768d1d0901ffffffff0200ca9a3b00000000434104ae1a62fe09c5f51b13905f07f06b99a2f7159b2225f374cd378d71302fa28414e7aab37397f554a7df5f142c21c1b7303b8a0626f1baded5c72a704f7e6cd84cac00286bee0000000043410411db93e1dcdb8a016b49840f8c53bc1eb68a382e97b1482ecad7b148a6909a5cb2e0eaddfb84ccf9744464f82e160bfa9b8b64f9d4c03f999b8643f656b412a3ac00000000
# Note: You need to set txindex=1 in bitcoin.conf to look up all the transactions in the blockchain.
This is useful when you want to check out the details of a transaction or to find out its location (i.e. if it has been mined into the blockchain or if it's still in the mempool).
2. Referencing previous outputs for spending
You use TXIDs for referencing outputs from previous transactions for use as inputs when you create a bitcoin transaction.
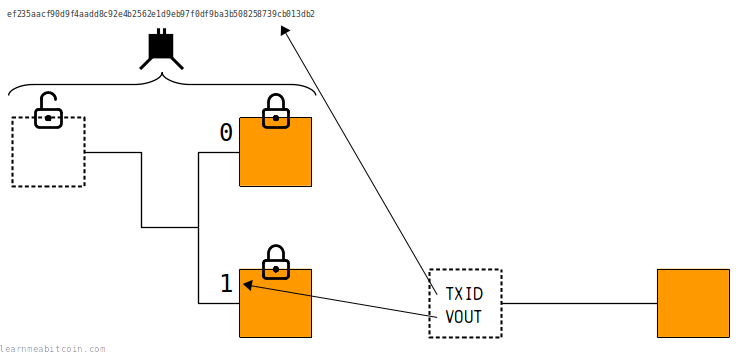
TXIDs are unique, so you can use them in combination with a VOUT to reference any specific output in the blockchain for spending.
3. Creating a merkle root
TXIDs are used to create the merkle root for the block header:
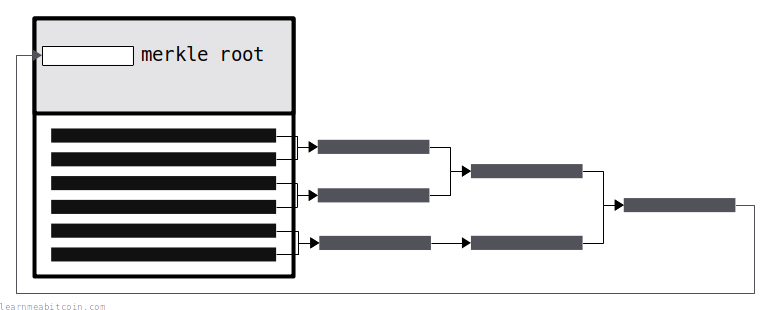
A merkle root is created by basically hashing all of TXIDs in a block in a tree-like structure. This creates a unique fingerprint for all the transactions inside the block, which then gets placed inside the block header to prevent the contents of the block from being tampered with later on.
This is because any change to transaction data will change the TXID, and any change to a TXID will have a knock-on affect to the resulting merkle root.