Address
An easy-to-share format of a locking script
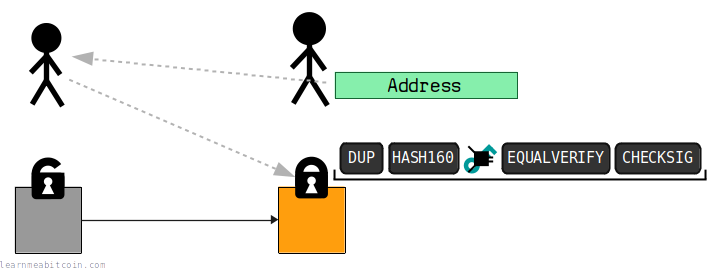
An address represents a specific type locking script to be placed on some bitcoins.
It's basically a user-friendly encoding of either a public key hash or a script hash.
So when you give someone an address, you're asking them to lock up your bitcoins to a specific script pattern using the public key hash or script hash contained inside the address.
Usage
How are addresses used in Bitcoin?
Addresses are used when you want to send bitcoins to someone using a bitcoin wallet.
When you make a transaction using a bitcoin wallet, the wallet will decode the address to determine the type of locking script pattern to place on the output, and extract the public key hash or script hash and place that inside the ScriptPubKey.
For example, a 1address contains a public key hash and corresponds to a P2PKH locking script:
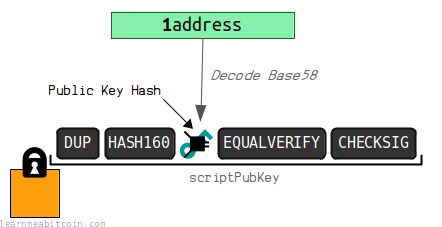
So addresses themselves do not appear inside the raw data in the blockchain – they are simply a user-friendly alternative to sending each other raw locking scripts.
Types
What are the different types of bitcoin addresses?
Different types of addresses correspond to different patterns of standard locking scripts.
Not all standard locking scripts have their own address. Only the most-commonly used locking scripts are assigned their own address format.
Do not use any of the addresses below. These are just examples, and you do not have the private key to be able to unlock any bitcoins sent to these addresses.
P2PKH
Base58
A 1address contains a public key hash and corresponds to a P2PKH locking script.
The address is a Base58Check encoding of the public key hash. This also includes a version byte prefix of 00
to force the first character of the address to be a 1, which helps distinguish it from a similar-looking P2SH address.
These addresses are case-sensitive.
P2SH
Base58
A 3address contains a script hash and corresponds to a P2SH locking script.
The address is a Base58Check encoding of the 20-byte script hash. This also includes a version byte prefix of 05
to force the first character of the address to be a 3, which helps distinguish it from a similar-looking P2PKH address.
These addresses are case-sensitive.
P2WPKH
Bech32
A 42-character bc1qaddress contains a public key hash and corresponds to a P2WPKH locking script.
The address is a Bech32 encoding of the full hex ScriptPubKey (which contains the public key hash). The address is always 42 characters in length, which helps to distinguish it from a similar-looking P2WSH address.
P2WSH
Bech32
A 62-character bc1qaddress contains a script hash and corresponds to a P2WSH locking script.
The address is a Bech32 encoding of the full hex ScriptPubKey (which contains the 32-byte script hash). The address is always 62 characters in length, which helps to distinguish it from a similar-looking P2WPKH address (which are 42 characters in length).
- The script hash for a P2WSH a 32-byte
sha256(script)
- The script hash for a legacy P2SH is a 20-byte
hash160(script)
P2TR
Bech32m
A 62-character bc1paddress contains a tweaked public key and corresponds to a P2TR locking script.
The address is a Bech32m encoding of the full hex ScriptPubKey. The address is always 62 characters in length and starts with bc1p, which helps to distinguish it from a similar-looking P2WSH address (which start with bc1q).
P2TR uses Bech32m encoding. This is a slight variation of the Bech32 encoding used for P2WPKH and P2WSH.
Purpose
Why do we use addresses in Bitcoin?
An address is a more convenient alternative to using raw locking scripts.
We could just send other people a complete locking script every time we want someone to "send" us some bitcoins, but this would be cumbersome, as the scripts would be unwieldy and mistakes are more likely to be made.
For example, if I wanted someone to send me some bitcoins, I could give them this complete locking script for them to use:
76a91404a621a724b8e064809d8fb22a65c2d37d01a01888ac
Alternatively, I could just give them this address instead:
1RainRzqJtJxHTngafpCejDLfYq2y4KBc
They both achieve the same result, but the address is shorter, more obvious, and easier to share.
An address is a short-hand way of writing locking scripts in a human-readable way.
Benefits
What are the features of bitcoin addresses?
There are a few benefits to using an address over a raw locking script:
- Error Detection. An address has a checksum built-in, which allows you to detect errors if the address has been input incorrectly, which helps to prevent you from sending bitcoins to an invalid address and losing the coins forever. This is the biggest advantage to using addresses, in my opinion.
- More Convenient. An address is shorter than a raw locking script and contains human-friendly characters (i.e. Base58, Bech32). This makes them easier to write down or share over the phone. It's not massively easier, but it is a slight improvement.
- Easily Identifiable. Addresses can be easily identified due to having standard character sets and structures. This helps you distinguish an address from other types of Bitcoin data. Furthermore, different types of addresses correspond to different types of locking scripts, so wallets (and humans, with some practice) can determine the type of lock that will be placed on an output by simply looking at the address.
So again, an address is simply a user-friendly alternative to using raw locking scripts when we want to send or receive bitcoins.
History
Addresses have always been a part of Bitcoin.
Base58 Addresses
2009 - present
Example Address | Script Type | Active |
---|---|---|
1RainRzqJtJxHTngafpCejDLfYq2y4KBc | P2PKH | 2009 - present |
3GTCwPn2EqSsAb3JDBo4WuwceVqkZjb83y | P2SH | 2012 - present |
The first 1addresses were designed by Satoshi for use with P2PKH locking scripts. Satoshi wanted to have a way for people to easily send and receive bitcoins, so they designed an address format that is the Base58check encoding of a public key hash. These always start with a 1.
Satoshi chose to encode a public key hash as opposed to a raw public key to create shorter addresses.
This style of Base58 format for addresses was continued with the introduction of 3addresses by Gavin Andresen in 2012 for use with P2SH locking scripts. These use the exact same Base58, but they contain a script hash, along with a 05
prefix to change the leading character of the address to a 3.
These are the "old-school" style of addresses, but they can still be used today.
Bech32 Addresses
2016 - present
Example Address | Script Type | Active |
---|---|---|
bc1q5twnm8gznrszgxw0t258ejxgz9nwda6fpfl0wh | P2WPKH | 2016 - present |
bc1qdj7v4lgweum6g8r8fjh5gedrwtg0pmf32uxnsvtf27adav96gftsudprkk | P2WSH | 2016 - present |
The new Bech32 address format was introduced in the Segregated Witness upgrade of 2016. It was designed by Pieter Wuille and Gregory Maxwell (YouTube Video).
The Bech32 address format improves upon the Base58 address format by using an improved checksum algorithm, and uses a case-insensitive set of 32 characters.
This address format was introduced for the new P2WPKH and P2WSH locking scripts. These work in essentially the same way as the original P2PKH and P2SH locking scripts they replace, but they use the Witness field instead of the ScriptSig field for unlocking.
The Witness field takes up less space inside a block than the ScriptSig, so transactions that spend these new P2WPKH and P2WSH scripts pay comparatively less in transaction fees.
These are the "modern" style of addresses and are preferable over the old-school Base58 addresses.
Bech32m Addresses
2021 - present
Example Address | Script Type | Active |
---|---|---|
bc1ppuxgmd6n4j73wdp688p08a8rte97dkn5n70r2ym6kgsw0v3c5ensrytduf | P2TR | 2021 - present |
Bech32m addresses are basically the same as Bech32 addresses.
The only difference is a slight change to the way the checksum is calculated. This is due to a weakness found in the original Bech32 encoding:
Bech32 has an unexpected weakness: whenever the final character is a 'p', inserting or deleting any number of 'q' characters immediately preceding it does not invalidate the checksum. This does not affect existing uses of [P2WPKH and P2WSH] addresses due to their restriction to two specific lengths, but may affect future uses and/or other applications using the Bech32 encoding.
So Bech32m addresses are visibly no different to Bech32 addresses, but their checksums are more reliable.
This address encoding is used for P2TR locking scripts, which were introduced in the Taproot upgrade in 2021.
Summary
I've said it many times already, but one more time for good measure: an address is just a user-friendly encoding of a locking script.
At first, you might think that an address is just an encoding of a public key, which is technically true to some degree, but to be more precise it's actually representing an instruction about the type and the contents of the locking script to be placed on an output.
This is because addresses can contain an encoding of any kind of data (e.g. script hash, public key hash, or tweaked public key) so they're not limited to locking outputs to public keys only.
If you're constructing raw transactions by hand then there's no need to use addresses, as they are not used internally in Bitcoin and do not appear in the blockchain. Instead, addresses are designed to be used externally by users when sending bitcoins via Bitcoin wallets.
Nonetheless, it's good to get to grips with the different types of addresses and the different types of locking scripts they refer to.